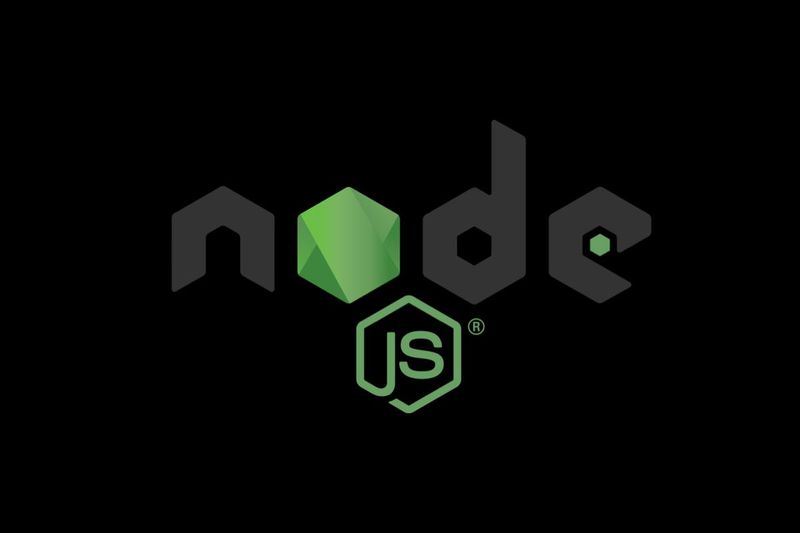
Express.js is a fast, minimal, and flexible Node.js web application framework that simplifies the process of building robust APIs and web applications. It provides a lightweight yet powerful structure to handle HTTP requests, middleware integration, and route management efficiently.
Installing and Setting Up Express
Before using Express.js, ensure that you have Node.js installed on your machine. You can verify this by running:
node -v npm -v
To install Express.js, follow these steps:
- Initialize a new Node.js project:
mkdir my-express-app && cd my-express-app npm init -y
- Install Express:
npm install express
- Create an
index.js
file and add the following code to set up a basic Express server:
const express = require('express'); const app = express(); const PORT = 3000; app.get('/', (req, res) => { res.send('Hello, Express!'); }); app.listen(PORT, () => { console.log(`Server running on http://localhost:${PORT}`); });
- Run the server:
node index.js
- Now, open
http://localhost:3000
in your browser to see the response.
Creating Routes in Express
Express makes it easy to define routes using HTTP methods such as GET, POST, PUT, and DELETE.
1. GET Request
app.get('/users', (req, res) => { res.send('Fetching all users'); });
2. POST Request
app.post('/users', (req, res) => { res.send('Creating a new user'); });
3. PUT Request
app.put('/users/:id', (req, res) => { res.send(`Updating user with ID ${req.params.id}`); });
4. DELETE Request
app.delete('/users/:id', (req, res) => { res.send(`Deleting user with ID ${req.params.id}`); });
Middleware in Express
Middleware functions in Express.js are used to process requests before they reach the final route handler. Middleware can be used for authentication, logging, parsing requests, and more.
Example of Middleware Usage
- Built-in Middleware:
app.use(express.json()); // Parses JSON request bodies
- Custom Middleware:
app.use((req, res, next) => { console.log(`${req.method} request made to ${req.url}`); next(); });
- Third-Party Middleware (Example:
morgan
for logging):
npm install morgan const morgan = require('morgan'); app.use(morgan('dev'));
Handling Errors and Debugging
Proper error handling is essential for debugging and maintaining an Express application.
1. Handling 404 Errors
app.use((req, res, next) => { res.status(404).send('Route not found'); });
2. Centralized Error Handling
app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Something went wrong!'); });
3. Using Debugging Tools
- Node.js Debugger: Run your app with
node --inspect index.js
. console.log()
Statements: Print logs at critical points.- Postman or cURL: Test API endpoints efficiently.
Conclusion
Express.js is a powerful yet simple framework that streamlines the process of building web applications and APIs. By understanding how to set up a project, create routes, use middleware, and handle errors, you can develop scalable and maintainable applications. Start experimenting with Express.js today to build dynamic and high-performance web applications!
Leave a Comment